Assembly Language
Greetings I had a question on assembly language I thought some of the people who are really into computers could help answer.
This is the code to create a horizontal line in the assembly language.
jmp CodeStart
DataStart:
xStart dw 50 ; x coordinate of line start
yStart dw 50 ; y coordinate of line start
length dw 25 ; length of line
CodeStart:
; set the video mode 320x200, 256 colors
mov al, 13h
mov ah, 0
int 10h
; initialize cx (x coord) to xStart + length
mov cx, xStart
add cx, length
; loop from (xStart+length) to xStart to draw a horizontal line
LoopStart:
; draw a pixel
; set color in al, x in cx, y in dx
mov al, 50
mov dx, yStart
; set sub function value in ah to draw a pixel
; and invoke the interrupt
mov ah, 0ch
int 10h
; decrement the x coord
sub cx, 1
; test to see if x coord has reached start value
cmp cx, xStart
; continue loop if cx >= xStart
jae LoopStart
ret
he wants me to make a vertical line with the same coordinates but going to 75 now is there an easier way to do this besides 2 loops or is there a way to get around the fact that the loopstart would be declared twice.
Last edited by Abangyarudo on 10 Dec 2008, 9:45 pm, edited 1 time in total.
... I don't think there was a specific one mentioned. We use the EMU8086 compiler if that helps any.
Golly gee, it might just be an 8086 processor!! !

Seriously, now ... what are the BIOS calls, if any, and what are the I/O and memory maps?
... I don't think there was a specific one mentioned. We use the EMU8086 compiler if that helps any.
Golly gee, it might just be an 8086 processor!! !

Seriously, now ... what are the BIOS calls, if any, and what are the I/O and memory maps?
shrugs I dunno its a beginning system architecture class and I dunno much about the processors besides what common people know.
I believe 10h since I was told to read the interupts in it and I'm not sure about the I/O. This is another part of the instructions.
Pay particular attention to subfunctions 00h and 0ch. Notice that by placing a value of 0 (zero) in the AH register and 13h in the AL register, we can create a video mode that is 320 pixels wide by 200 pixels high.
To draw single pixel, we can use subfunction 0ch. When using this interrupt, we put the pixel x value in the CX register, the pixel y value in the DX register and the pixel color in the AL register.
The following code segment sets the video mode and draws a few pixels:
; set the video mode 320x200, 256 colors
mov al, 13h
mov ah, 0
int 10h
; draw a pixel
; set color in al, x in cx, y in dx
mov al, 50
mov cx, 20
mov dx, 10
mov ah, 0ch
int 10h
; draw some more pixels
mov al, 50
mov cx, 21
mov dx, 10
mov ah, 0ch
int 10h
mov al, 50
mov cx, 22
mov dx, 10
mov ah, 0ch
int 10h
Okay ... I'm gonna level with you. Try to not take it as an insult, but you really need to study the programming environment instead of trying to use someone else to provide the answers for you.
Those questions I asked ... processor, BIOS, I/O and memory maps ... they're all important. At the very least, you need to know the brand name of the computer you're using, as well as the operating system.
Those questions I asked ... processor, BIOS, I/O and memory maps ... they're all important. At the very least, you need to know the brand name of the computer you're using, as well as the operating system.
this class is really just designed to get down the basics we don't discuss what they are for and hence don't get into what I/O we are using the bios I'm not sure which it is. So I will go ahead and try but I was just looking to see if theres a way to do it better since right now I have 2 loops with 1 loop pretty much reversing whats done in the other loop the problem is it skips the 2nd loop start and if I have it direct to both it does both functions once instead of comparing them to the x and y start. The 2nd loop just gets ignored and this is due soon because finals are soon and I have to get this in by friday.
Now if I do it with the two loops I get an error for having two declarations but if I renane loop start and have it cross between the two loops it goes through each 1 (effectively drawing 2 green dots) which if I am reading correctly means that its only processing each loop once.
So either I need a command which will process the 2nd loop after the first or I need a way to process both loops efficently (which my current method is really not that great on efficency). It sucks cause my one and a half week away due to moving and stuff put me so far behind.
You are going to be using the bios constantly. You need to know what that is, what it does.
mov al, 13h means move the hex(h) value 13 into the al register. AX stands for accumulator, and is used mostly for math. AL is the low end of itm AH is the high end. And right there the processor type is important as different machines read the numbers oppositely.
int 10h considers the value of AX(AL and AH) as an instruction or setting value. int stands for interrupt, which is a call to the processor to do something. In this case, to change the graphics mode. Interrupts speak to the processor and tells it to activate micro code, which is vaguely like a tiny operating system built into the processor. Again, you can see why you need to know details of the computer.
A) you are not quite ready for this class.(but its fairly easily rectifiable).
B) I think your teacher probably sucks.
These are called registers.
AX = accumulator pointer - math
BX = base pointer - general purposes
CX = counter pointer
DX = data pointer
each one is split into two, so you have AH,AL, BH, BL, CH....
and each can be considered as one, or as halves depending on usage.
_________________
davidred wrote...
I installed Ubuntu once and it completely destroyed my paying relationship with Microsoft.
mov al, 13h means move the hex(h) value 13 into the al register. AX stands for accumulator, and is used mostly for math. AL is the low end of itm AH is the high end. And right there the processor type is important as different machines read the numbers oppositely.
int 10h considers the value of AX(AL and AH) as an instruction or setting value. int stands for interrupt, which is a call to the processor to do something. In this case, to change the graphics mode. Interrupts speak to the processor and tells it to activate micro code, which is vaguely like a tiny operating system built into the processor. Again, you can see why you need to know details of the computer.
A) you are not quite ready for this class.(but its fairly easily rectifiable).
B) I think your teacher probably sucks.
These are called registers.
AX = accumulator pointer - math
BX = base pointer - general purposes
CX = counter pointer
DX = data pointer
each one is split into two, so you have AH,AL, BH, BL, CH....
and each can be considered as one, or as halves depending on usage.
yea you should probably be teaching the class I do software programming ok with the easier languages but hardware programming has been really difficult. I am not completely sure the real value of this course in my degree program as it is more for console design then game design. I think its just more of the teacher doesn't really seem to explain things to the students who are confused as he has received complaints from students. (alot of the students who live around a campus acutally just go for the tutoring.) Since mostly everyone who is doing good in the class has previous experience. So in regards to the registers (since we been mainly just using registers as storage places without much regard to the specifics of each type)
I think honestly I will be retaking the course at another school since I dislike only half learning something and since I have alot of problems with the language.
Those questions I asked ... processor, BIOS, I/O and memory maps ... they're all important. At the very least, you need to know the brand name of the computer you're using, as well as the operating system.
just to clarify something incase you got the wrong idea this is lab 6 out of 7 I've done 5 of them already without that information. So incase you thought this was my first time with the class and I was running to other people then your wrong. Essentially how I am approaching this is not working so I was looking into ways I can improve what I have and fix the problems with it skipping the loop.
This class is the bare basics as you can see though I know what a I/O is we don't discuss the targets of the labs we just create,modify, or restructure code within the compiler. While I have read all the coursework which sadly enough didn't even have the different type of registers like the poster above provided me with. So while I'm not claiming to know about the bios and other factors I really don't see myself doing hardware programming as since I am going for a game degree and most of my work is in engines (Torque, Unreal 2 etc) mostly simple 2d rpgs and stuff.
Right now my focus is on just passing the class (my grades have dropped from a 85 to a 60 due to him giving me zeros for work I was supposed to complete but couldn't due to shipping my computer when I moved it got lost and took longer then it should have). Really I'm just looking for better ways to accomplish this, as we can see with the teacher I have and the bare course essentials it won't happen this semster but I'm looking to pinpoint the problem and anything I learn in the process is cool. So if I do bad I do bad as right now I'm fighting over the grades with the school because of all the marked 0's when it defies the late policy which gives me an extra week.
Those questions I asked ... processor, BIOS, I/O and memory maps ... they're all important. At the very least, you need to know the brand name of the computer you're using, as well as the operating system.
Those questions you asked were all completely irrelevant.
The code is obviously for an 8086 (or upward).
The BIOS calls are exactly shown, and functional.
There is no I/O (other than shown).
There is no memory map involved.
There is no brand name.
There is no operating system.
==============
And, to answer the OP's questions...
I think he is asking you to adapt the code to draw a vertical, instead of horizontal line... not do both. I.e. you will end op with almost exactly the same code - one loop, using that same label "loopstart".
To go vertical, instead of horizontal, you need to make the Y-coordinate vary. That means the value passed in register "dx" is what needs to be varied.
So, you need to transpose the handling that involves "cx" with that using "dx", along with swapping the "x" and "y" stuff, and changing "length" to have 75.
You should end up with exactly the same set of instructions, just with addresses and registers switched about a little.
_________________
"Striking up conversations with strangers is an autistic person's version of extreme sports." Kamran Nazeer
This is the code to create a horizontal line in the assembly language.
....
he wants me to make a vertical line with the same coordinates but going to 75 now is there an easier way to do this besides 2 loops or is there a way to get around the fact that the loopstart would be declared twice.
First off, your horizontal line algorithm lacks palette initialization. You cannot write dependable 256 color code without palette initialization. The colors will be random or not present if you try. The COLOR input to the set_dot bios interrupt is not actually a color, but rather a palette index. The palette is where the colors are. The palette array has the RGB values associated with a particular color. If you go to 15, 16 or 32 bit mode, you are writing RGB information directly to the screen memory. But as far as the palette initialization, if you don't do it in 256 color mode, your palette is whatever the last program that used it set it to. In your example, you only need to set two colors and not all 256 since that is all you are using.
As far as your horizontal line, I don't see anything glaring as to why it wouldn't work other than the palette issue. For a vertical line, its really simple. Try this psuedocode algorithm:
Init_256_color_Video_mode(); // this is 320 wide by 200 tall
Init_Palette();
X=100;
Y=10;
Len=75; // Note that this will NOT work if Len = 0;
loop:
Set_Dot(X, Y);
Y = Y+1;
Len = Len - 1;
If (Len != 0) goto Loop:
Done:
The above code would draw a vertical line from 100,10 to 100, 85.
Those questions I asked ... processor, BIOS, I/O and memory maps ... they're all important. At the very least, you need to know the brand name of the computer you're using, as well as the operating system.
Those questions you asked were all completely irrelevant.
The code is obviously for an 8086 (or upward).
The BIOS calls are exactly shown, and functional.
There is no I/O (other than shown).
There is no memory map involved.
There is no brand name.
There is no operating system.
==============
And, to answer the OP's questions...
I think he is asking you to adapt the code to draw a vertical, instead of horizontal line... not do both. I.e. you will end op with almost exactly the same code - one loop, using that same label "loopstart".
To go vertical, instead of horizontal, you need to make the Y-coordinate vary. That means the value passed in register "dx" is what needs to be varied.
So, you need to transpose the handling that involves "cx" with that using "dx", along with swapping the "x" and "y" stuff, and changing "length" to have 75.
You should end up with exactly the same set of instructions, just with addresses and registers switched about a little.
sorry if I declared wrong but he said he wanted a vertical line in addition to the horizontal one., What I did was make two loops cause how its coded it keeps drawing the line until its equal to the length (I know you probably understand that but to reiterate) when I do the two loops it doesn't perform that function. It does a dot each which means the loops were only ran once each. Thanks in advance for your suggestions.
Last edited by Abangyarudo on 11 Dec 2008, 12:51 am, edited 2 times in total.
This is the code to create a horizontal line in the assembly language.
....
he wants me to make a vertical line with the same coordinates but going to 75 now is there an easier way to do this besides 2 loops or is there a way to get around the fact that the loopstart would be declared twice.
First off, your horizontal line algorithm lacks palette initialization. You cannot write dependable 256 color code without palette initialization. The colors will be random or not present if you try. The COLOR input to the set_dot bios interrupt is not actually a color, but rather a palette index. The palette is where the colors are. The palette array has the RGB values associated with a particular color. If you go to 15, 16 or 32 bit mode, you are writing RGB information directly to the screen memory. But as far as the palette initialization, if you don't do it in 256 color mode, your palette is whatever the last program that used it set it to. In your example, you only need to set two colors and not all 256 since that is all you are using.
As far as your horizontal line, I don't see anything glaring as to why it wouldn't work other than the palette issue. For a vertical line, its really simple. Try this psuedocode algorithm:
Init_256_color_Video_mode(); // this is 320 wide by 200 tall
Init_Palette();
X=100;
Y=10;
Len=75; // Note that this will NOT work if Len = 0;
loop:
Set_Dot(X, Y);
Y = Y+1;
Len = Len - 1;
If (Len != 0) goto Loop:
Done:
The above code would draw a vertical line from 100,10 to 100, 85.
ok thank you after I try the other posters suggestions I will try researching palette initialization. with this is it needed for a screen that is black with green text assuming it would follow that pattern again which is all I need for the program unless I'm really misunderstanding you.
ValMikeSmith
Veteran
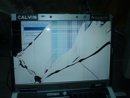
Joined: 18 May 2008
Age: 54
Gender: Male
Posts: 977
Location: Stranger in a strange land
Cool that you are learning game programming, it rules!
Assembly Language is ALWAYS the fastest.
Assembly Language ALWAYS takes the least amount of memory.
It makes Windows look like the rubbish heap of code that Windows really is.
For mind-blowing game programming inspiration, try running some "Demoscene" code.
If you have a PC, I recommend my favorite, Google "Farbrausch fr-08",
which does almost impossible amazing graphics with only about 64000 bytes of code!
Assembly Language is ALWAYS the fastest.
Assembly Language ALWAYS takes the least amount of memory.
It makes Windows look like the rubbish heap of code that Windows really is.
For mind-blowing game programming inspiration, try running some "Demoscene" code.
If you have a PC, I recommend my favorite, Google "Farbrausch fr-08",
which does almost impossible amazing graphics with only about 64000 bytes of code!
wow I will check that out when I'm not behind in school work