Developing a forum from scratch?
Hi!
So, Im developing a website from scratch for a friend. This website im making for him is targeted at Uni students so it would need to be very secure. My PHP skills are pretty good but my hacking skills are not. My friend wants this site to be uber simple and easy on the eye. The site is basically a very very light forum. Its tricky to explain but il try and put it best I can.
Heres the flow:
When you log in you are introduced with a home screen. This home screen has a post a question button along with a view questions button. When you press either the view questions or create question button you are then introduced to a screen which asks you which category you belong to. So lets say if I belonged to category 1, id select category 1 to post or view a question to. When you submit a question you can dish out rewards. You chose the reward amount and the user you press as the correct answer gets the reward.
I would be using MyBB but this forum will have an awards system which would definitely need to be customised and programmed by me. To give you an idea how good I am at programming, I am 16. I started with doing Visual Basic when I was about 8 and then when I was 12 I started on PHP, HTML, CSS and Objective C. Since then I have actually programmed my own forums (this was when I was 14) from scratch which worked well but never published. So... Basically, would it be secure enough if I made sure to look out for injections? What do I have to look out for?
Thanks!
_________________
?Sometimes when you innovate, you make mistakes. It is best to admit them quickly, and get on with improving your other innovations.? -Steve Jobs.
Disclaimer: I got my start playing with platforms and have since moved through mobile and towards web development...
Injections are quite common difficulties for basically any framework, but not so much as automated exploits. Considering my cursory reading of the exploits du jour, multi factor authentication probably ranks about the same in terms of importance. A Wordpress install I'm currently working to republish shows no signs of compromise in the zipped backup and the conclusion from the inaccessibility of the server from most clients is that the host itself was compromised, most likely centrally or from a brute-forced control panel. There are many ways of implementing multi factor logins, the best of which tend to involve a separate device, but since you're building this for students it seems risky to assume they have constant access to anything but laptops. Although you COULD build your 2 factor login in automation script around USB drives, I think expiring logins with email or SMS tokens would do the trick, perhaps you could go through a service such as SendGrid to distribute these, and I also think SMS is faster. I'd rather pick my phone up than login to one of my zillion email accounts, if you need an example of this, Gmail recently adopted it.
_________________
"Standing on a well-chilled cinder, we see the fading of the suns, and try to recall the vanished brilliance of the origin of the worlds."
-Georges Lemaitre
"I fly through hyperspace, in my green computer interface"
-Gem Tos

A basic rule is to never, EVER trust any user input. Always use things like prepared statements when querying the database and always filter any user input when you use it in the output of a webpage.
For my understanding, are you making something similar to stackoverflow? People post a question about various topics and the answers other people give get upvoted, or awarded points? If so, you may want to steal some of their ideas . Perhaps there's even opensource projects for things similar to stackoverflow.
By developing something from scratch you're pretty safe from automated attacks from bots. Obviously the other side is that you have to put in all the work of making it safe yourself, which depending on your level of experience could be hard.
For my understanding, are you making something similar to stackoverflow? People post a question about various topics and the answers other people give get upvoted, or awarded points? If so, you may want to steal some of their ideas

By developing something from scratch you're pretty safe from automated attacks from bots. Obviously the other side is that you have to put in all the work of making it safe yourself, which depending on your level of experience could be hard.
Set up a temporary localhost domain with your favorite tool, then tear your work to pieces using Kali Linux and/or Backtrack pentester's Tools. Not something I've had to do myself, but I enjoy possessing the capability. I'm pretty sure you can even choose to run these distros through KDE.
_________________
"Standing on a well-chilled cinder, we see the fading of the suns, and try to recall the vanished brilliance of the origin of the worlds."
-Georges Lemaitre
"I fly through hyperspace, in my green computer interface"
-Gem Tos

sliqua-jcooter
Veteran
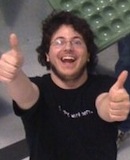
Joined: 25 Jan 2010
Age: 37
Gender: Male
Posts: 1,488
Location: Burke, Virginia, USA
Bottom line: You don't know what you don't know. You can't guard against unknown vulnerabilities - so you have to find out what your application could be vulnerable to. In other words, fuzz the crap out of it.
_________________
Nothing posted here should be construed as the opinion or position of my company, or an official position of WrongPlanet in any way, unless specifically mentioned.
Indeed. Backtrack contains quite a number of high-level automated tools for doing just this. It takes a lot of shell input, but you can just generate some script to repeat & iterate your process.
_________________
"Standing on a well-chilled cinder, we see the fading of the suns, and try to recall the vanished brilliance of the origin of the worlds."
-Georges Lemaitre
"I fly through hyperspace, in my green computer interface"
-Gem Tos

Ok there are several tricks you could use to prevent a injection using PHP.
Here is what I do:
$temp = strlen($_POST['value_from_form']);
#if value of temp is greater than my known table value I am looking for ie username has a max length of 35
if($temp >= 35){
#send them somewhere else
header( 'Location: some website' ) ;
}
#lets add some more security
$temp = $_POST['value_from_form']
$queryItem = mysql_real_escape_string($temp)
#the above escapes any quotes that may be added by adding a \ to the string if it finds any (doesn't hurt your MySql query at all)
Just some ideas on how to sure it up, I'm sure with your experience you can come up with more.
Also I would never ever put your query on your action page. Use a custom class that is outside your root folder and give your script permission to read it. Also never ever place your username, password, dbname, or dbtable name directly in a php file. Place it again outside of root in a txt or xml file that needs to be read by your class that isn't accessible to anyone accessing the site from the internet.
After that you have the server and MySQL functions you can also use to sure up you app/site, but just remember NOTHING is totally secure so keep that in mind when storing information that you don't want others to have. (Separating info into several tables is one trick if you really need to have a lot of personal info and never name your tables based on the information stored. For example never name a table User_tbl or any variation of it name it instead My_Go_To_Mess_tbl or something not obvious.)
You should know that the mysql_* extension is deprecated now and will be removed in a future version of PHP. You could use mysqli as the methods are pretty similar so the switch might be easier. Or you could go with PDO and become more flexible with which database system you use.
Splitting information across tables with hard to guess names will IMO only lead to a hard to maintain system and it only really offers security through obscurity. Depending on how much data is being stored such an approach can also kill performance as you'll have to JOIN serveral different tables.
@OP:
Oh, and remember to hash and salt passwords!
I haven't programed in PHP since 2009 so I am sorry I gave dated info, been busy with other languages and technologies not web server related.
I am going to preface this with that I respectfully disagree with rickith. I also apologize for not being more clear, I just expect people to think like I do and come to some logical conclusions on their own based on a overly simplified example rather than having to spell it out. Sorry ( EDITED PART: I'm working on it, but it's one of those black or white things. Hopefully this will be somewhere in the middle.)
Keeping it simple because your afraid of hard to maintain db in my opinion is a sign that there is no documentation, something that should always be done as well as being poor db planning. Data should be separated as to it's function and use. You won't be calling for ALL the user's information ALL the time. That would put an unneeded strain on your server.
For example let's just create a very simple db schematic for a user of a forum like this one.
I would split it up like so:
Table name: MyWebsite_Privy_Intelligence
Columns: PIN_AvatarLocation, PIN_Diagnosis, PIN_ID (primary key), PIN_Location, PIN_PersonalLoginID (aka PB_ID), etc
Table Name: MyWebsite_Privy_bootUp
Columns: PB_ID (primary key also used as identifier in all personal info tables), PB_Password, PB_User
Table Name: MyWebsite_Privy_Inquery
Columns: PI_ID(pk), PI_PersonalLoginID(aka PB_ID), PI_ID(aka QI_ID), PI_Answer
Table Name: MyWebsite_InQueryIdentifier (if you want them to create their own personal security questions makes it harder for hackers to guess)*see note below
Columns: IQI_ID(pk), IQI_PersonalLoginID(aka PB_ID), IQI_Question
Notice each table has an obscure name but not unidentifiable they are Synonyms for what each table holds. Privy = Personal, bootup = login, Inquery = question, intelligence = information
You would split up the forum information the same way. Categories, Subcategories, Original Posts, Replies (or something along those lines)
This allows for more flexibility to changes in your server code and keeps your logic, data, and display distinct and secure. Plus it is a relational db schematic something much easier to read when you have large volumes of information.
As far as JOIN statements, you should be using stored procedures so that your web server isn't doing the work your db server should be doing and they should be separate machines for security reasons.
Though I do agree your passwords should be hashed. There is a ton you can do to secure anything, but only if you are willing to put the time and effort into it. AND REMEMBER TO DOCUMENT!
*note: Asking a pet name is fairly standard and if you are familiar with dog names I can ramble off at least 5 common names that people name their dogs. spot, rex, buster, sam, rocky, cody, buddy, buffy, princess, max.....you get the idea. So allowing them to come up with their own or at least not asking common questions (ie: What is your cousins middle name?) would be better.
Maybe just us this phpBB and mysql_real_escape.
Most important use git and regular backups to some safe not accessible from outside place.
So you may notice if you were hacked. It will save you lots of time.
I used to hack things 6 years ago when I only started to learn php, I coded with C++ and Assembler before php, cracked some simple programs with hex editor and w32dasm.
Once I hacked a forum and contacted system administrator, explained how I hacked it and we became good friends.
So in your case if some student hacks your forum you may try to friend him.
+ backup will restore all points back.
Similar Topics | |
---|---|
New to the forum and the reality of ASD |
02 Jan 2025, 7:01 pm |
forum post likely to increase polarization |
28 Dec 2024, 12:54 pm |
Finally managed to join the forum! |
21 Jan 2025, 11:30 pm |